Handling Strings in Python
A string is a collection of characters enclosed in single quotes ('
), double quotes ("
), or triple quotes ('''
or """
). While triple quotes are often used for multiline strings, Python treats single characters as strings with a length of 1 since it does not have a separate character data type.
Accessing Characters in a String
Strings are collections of characters, and each character can be accessed using its index. Python indexing starts from:
0
for the first character (forward indexing).-1
for the last character (backward indexing).
Visual Representation of Indexing
To better understand indexing, refer to the image below:
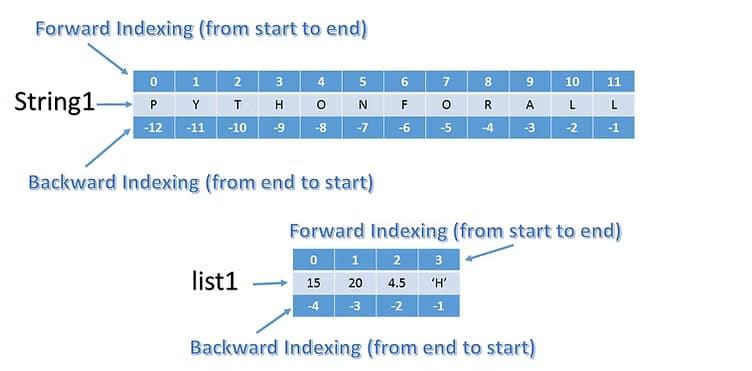
Example:
String1 = "PYTHONFORALL"
# Accessing characters using forward indexing
print(String1[3]) # Output: H
# Accessing characters using backward indexing
print(String1[-3]) # Output: A
Important Note:
Providing an index out of range will result in an IndexError
.
Escape Sequences in Strings
Escape sequences allow you to include special characters in strings that cannot be directly typed. They begin with a backslash (\
).
Escape Sequence | Description | Example | Output |
---|---|---|---|
\' | Single Quote | 'Python\'s book' | Python's book |
\" | Double Quote | "Python says \"Hi!\"" | Python says "Hi!" |
\\ | Backslash | "Path: C:\\Program" | Path: C:\Program |
\n | Newline | "Hello\nWorld" | Hello World |
\t | Tab | "Hello\tWorld" | Hello World |
\b | Backspace | "Helloo\b" | Hello |
Example:
# Using escape sequences
print("Python says \"Learn Strings!\"")
print("Path: C:\\Users\\YourName")
print("Hello\nWorld")
String Operations
1. Concatenation
Concatenation is the process of joining two strings using the +
operator.
Example:
name = "Rhea"
msg = "Hello "
print(msg + name) # Output: Hello Rhea
2. Repetition/Replication
Replication repeats the string n
times using the *
operator.
Example:
name = "PythonForAll"
print(name * 4) # Output: PythonForAllPythonForAllPythonForAllPythonForAll
3. Slicing
Slicing extracts a substring from the main string based on specified index values.
Example:
name = "PythonForAll"
# Slice using forward indexing
print(name[0:6]) # Output: Python
# Slice using backward indexing
print(name[-6:-2]) # Output: ForA
# Slice with step value
print(name[1:8:2]) # Output: yhno
# Reverse the string
print(name[::-1]) # Output: llAroFnohtyP
Membership Operators in Strings
Python uses in
and not in
operators to check for the presence of substrings in a string.
Example:
name = "PythonForAll"
# Using 'in'
print("on" in name) # Output: True
# Using 'not in'
print("All" not in name) # Output: True
Built-In String Functions
Python provides several built-in functions to manipulate strings. Here are some commonly used ones:
Function | Description | Example | Output |
---|---|---|---|
upper() | Converts the string to uppercase | "hello".upper() | "HELLO" |
lower() | Converts the string to lowercase | "HELLO".lower() | "hello" |
swapcase() | Toggles the case of each character | "PyThOn".swapcase() | "pYtHoN" |
startswith() | Checks if string starts with a substring | "hello".startswith("he") | True |
endswith() | Checks if string ends with a substring | "hello".endswith("lo") | True |
find() | Finds the first occurrence of a substring | "hello".find("e") | 1 |
replace() | Replaces occurrences of a substring | "hello".replace("l", "z") | "hezzo" |
len() | Returns the length of the string | len("hello") | 5 |
isalpha() | Checks if all characters are alphabets | "hello".isalpha() | True |
isdigit() | Checks if all characters are digits | "1234".isdigit() | True |
strip() | Removes whitespace from both ends of the string | " hello ".strip() | "hello" |
split() | Splits a string into a list of substrings | "Python For All".split() | ["Python", "For", "All"] |
Examples
Example 1: Menu-Based String Manipulation
# Menu-Based String Manipulation
option = int(input("Choose an option (1: Uppercase, 2: Lowercase): "))
text = input("Enter a string: ")
if option == 1:
print("Uppercase:", text.upper())
elif option == 2:
print("Lowercase:", text.lower())
else:
print("Invalid option")
Example 2: Toggle Case
# Toggle Case
text = input("Enter a string: ")
print("Toggled Case:", text.swapcase())
Example 3: Check Palindrome
# Check Palindrome (Without Slicing)
text = input("Enter a string: ")
reversed_text = ''.join(reversed(text))
if text == reversed_text:
print(text, "is a palindrome")
else:
print(text, "is not a palindrome")
Try It Yourself
Problem 1: Count Vowels
Write a program to input a string and count the number of vowels (a, e, i, o, u
) in it.
Show Code
text = input("Enter a string: ")
vowels = "aeiouAEIOU"
count = 0
for char in text:
if char in vowels:
count += 1
print("Number of vowels:", count)
Problem 2: Replace Substring
Write a program to input a string and replace all spaces with underscores (_
).
Show Code
text = input("Enter a string: ")
print("Modified string:", text.replace(" ", "_"))
Problem 3: Reverse a String
Write a program to input a string and print it in reverse order.
Show Code
text = input("Enter a string: ")
print("Reversed string:", text[::-1])