Flow of Control in Python
Flow of control refers to the order in which individual statements, instructions, or functions are executed in a program. Python provides three basic types of control structures to determine the flow of execution: Sequential, Conditional, and Iterative.
1. Sequential Flow
Sequential flow is the default mode of execution in Python, where statements are executed one after another in the order they appear.
Characteristics:
- No decision-making or repetition.
- Each statement runs exactly once.
Example:
# Sequential Execution
print("Step 1: Start")
print("Step 2: Process")
print("Step 3: End")
Flowchart:
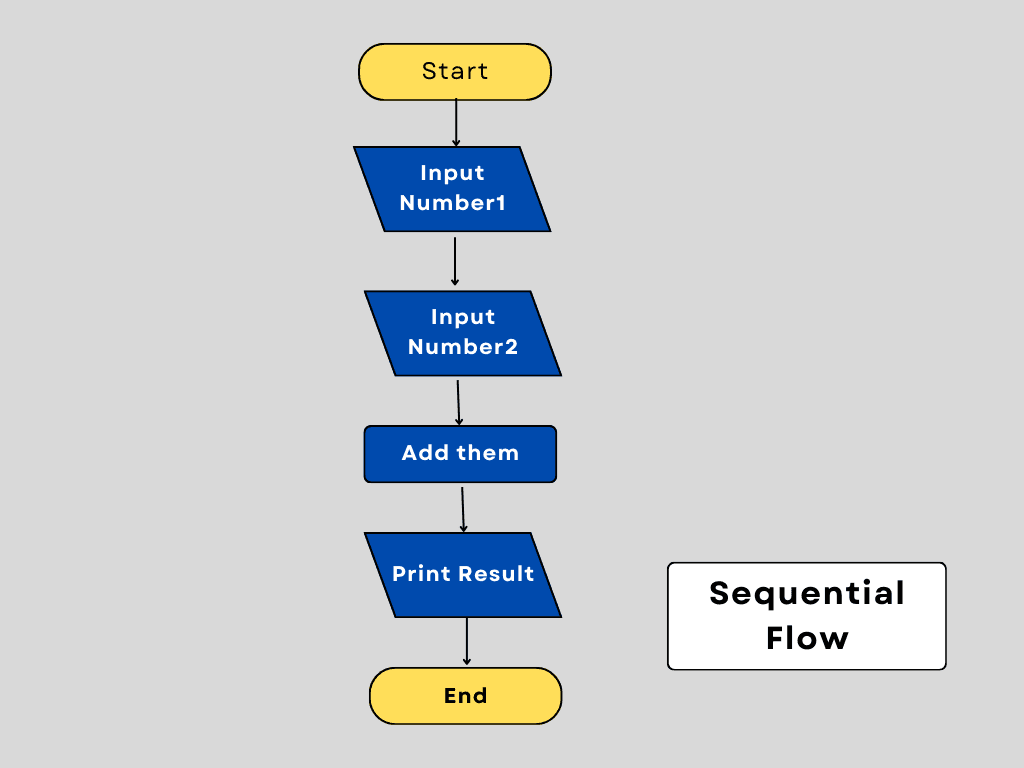
2. Conditional Flow
Conditional flow allows decision-making in a program. The flow of execution depends on whether a specified condition evaluates to True
or False
.
Types of Conditional Statements:
if
Statement: Executes a block of code if the condition is true.if-else
Statement: Executes one block if the condition is true, another if it is false.if-elif-else
Statement: Tests multiple conditions.
Example:
# Conditional Flow
marks = 85
if marks >= 90:
print("Grade: A+")
elif marks >= 75:
print("Grade: A")
else:
print("Grade: B")
Flowchart:
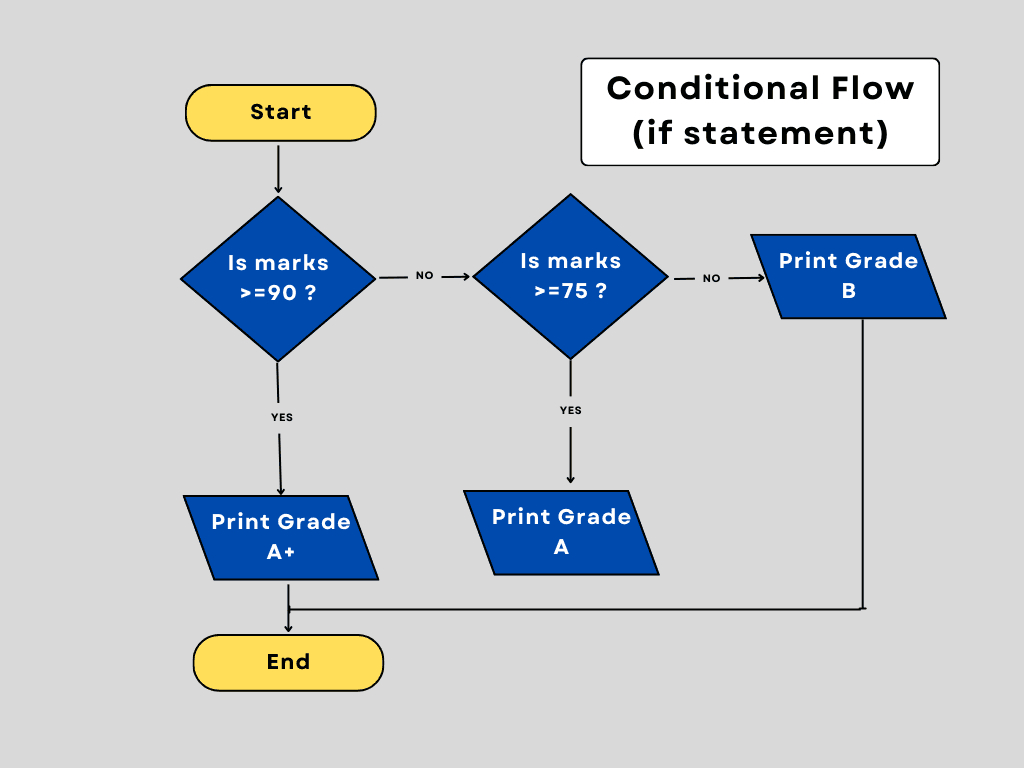
3. Iterative Flow (Loops)
Iteration allows the repetition of a block of code multiple times, as long as a specified condition holds true. Python supports two main types of loops:
for
Loop: Used to iterate over a sequence (like a list or string).while
Loop: Repeats as long as a condition is true.
Example:
# Iterative Flow using a while loop
counter = 1
while counter <= 5:
print("Count:", counter)
counter += 1
Flowchart:
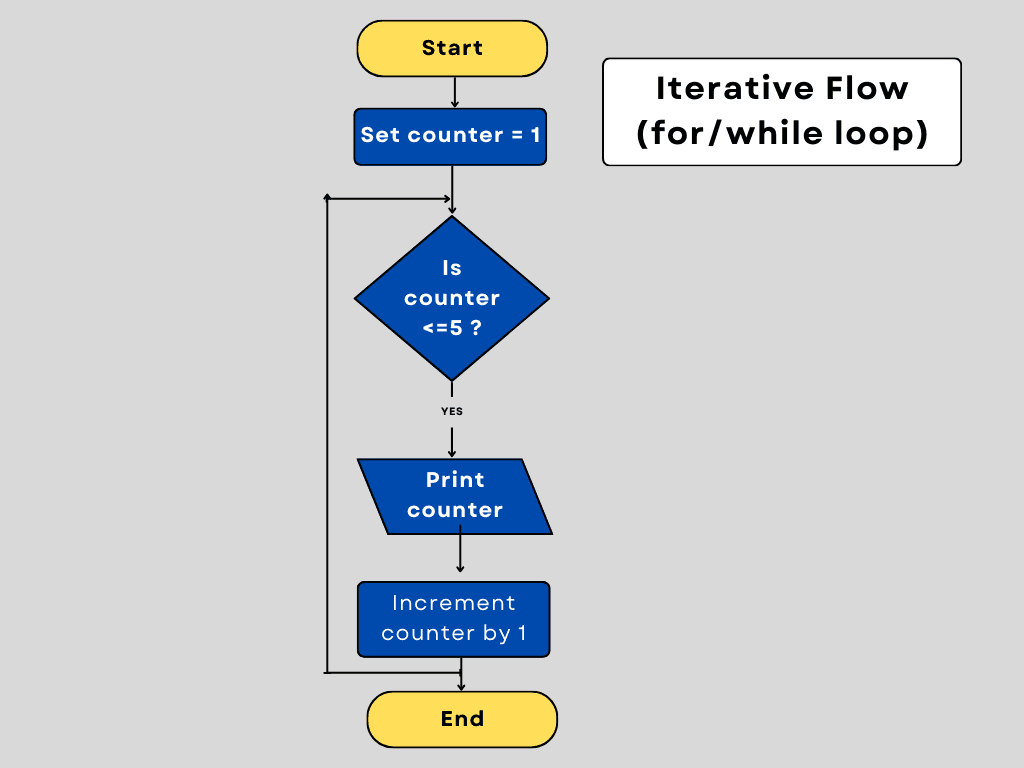
Combining All Flows
In real-world scenarios, programs often combine all three types of control flows:
- Sequential: For regular steps like initialization and cleanup.
- Conditional: For decision-making based on user inputs or results.
- Iteration: For repetitive tasks like processing data.
Example:
# Combining Sequential, Conditional, and Iterative Flows
print("Welcome to PythonForAll")
marks = int(input("Enter your marks: "))
if marks >= 50:
print("You passed")
for i in range(3):
print("Congratulations")
else:
print("Better luck next time")
Summary of Flow of Control
Type | Description | Example |
---|---|---|
Sequential | Statements executed one after another. | print("Hello") followed by print("Bye") |
Conditional | Executes code based on conditions. | if x > 0: print("Positive") |
Iterative | Repeats code multiple times. | for i in range(5): print(i) |