TKINTER MODULE
Python provides a variety of options for GUI development (Graphical User Interface). Tkinter is the approach used the most frequently among all GUI approaches. It is a typical Python interface for the Python-supplied Tk GUI toolkit. The fastest and simplest way to create GUI applications is with Python and Tkinter. Tkinter makes building a GUI a simple process.
For installing Tkinter Module :
Use "pip install tkinter"
For importing Tkinter Module :
Use "import tkinter as tk"
Tkinter Basics
Creating Window
For making any GUI Interface the 1st Basic Step is to create a Window :
Syntax : window = tk.Tk()
FUNCTIONS :-
-
To Name your window
>> window.title("Tkinter Window Demo")
-
To Give size to your window (200= width ,300= height)("+100" is used to change the position of window on Y-Axis & "+500" for X-Axis)
>> window.geometry("200x300+100+500")
-
To Make User unable to resize the window
>> window.resizable(False, False)
-
To Make the window run in a loop so that it wont get closed
>> window.mainloop()
-
To Make the window run for limited time (5000= 5 Seconds/5000 Milliseconds)
>> window.after( 5000, window.destroy )
Adding Widgets
After creating a window, The next step is to add widgets to your window which improves the functionality of your GUI Program.
There are many Widgets that are provided by Tkinter :-
FRAME
Tkinter Frame widget is a container that holds other Tkinter widgets like Label, Entry, Listbox, etc.
Syntax : frm1=Frame(parentwindow, parameters)
Example :-
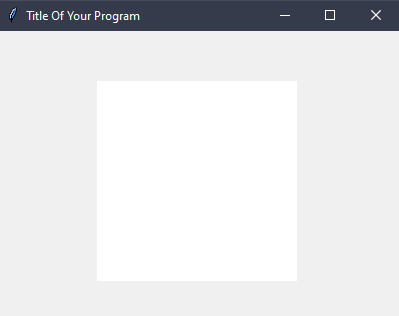
LABEL
Tkinter Label widget is just like a text box, we can use it as a container to place text or images.
Syntax : lbl1=Label(parentwindow, parameters)
Example :-

CANVAS
Tkinter Canvas widget lets us draw figures or shapes as the name suggests, we can use it as a sketchpad.
Syntax : Cvs1=Canvas(parentwindow, parameters)
Example :-

ENTRY
Tkinter Entry widget is similar to the Input function of Core Python, we can use it to let the user enter any string/int/float value.
Syntax : Ent1=Entry(parentwindow, parameters)
Example :-
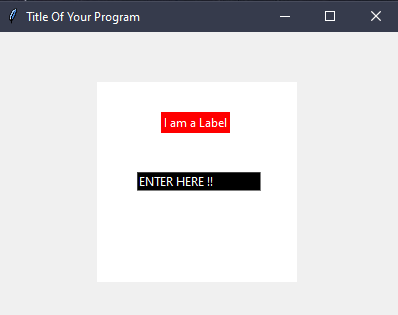
BUTTON
Tkinter Button widget is same as the name suggests a button which can be given a command to perform when pressed.
Syntax : Btn1=Button(parentwindow, parameters)
Example :-

MENU
Tkinter Menu widget is an OptionMenu which have options like Save , New , Open , Save as.. , etc.
Syntax : Men1=Menu(parentwindow, parameters)
TOP LEVEL
Tkinter TopLevel is a widget used to make a New Window aside from the Parent Window.
Syntax : NewWin=Toplevel(parameters)
MESSAGE BOX
Tkinter Messagebox is a widget used to generate & display a message box on a particular command.
Syntax : messagebox.Function_Name(title, message , parameters)
For further information, please read the official document of Tkinter Module. Click Here!
Placing Widgets
After creating a widget, The next step is to place widgets to your window which helps to display the widgets properly in your GUI Program.
There are 3 Ways to place your Widgets that are provided by Tkinter :-
Pack
Pack is the easiest layout manager to use with Tkinter , Instead of declaring the precise location of a widget, pack() declares the positioning of widgets in relation to each other. However, pack() is limited in precision compared to place() and grid() which feature absolute positioning.
Syntax : widget_name.pack(parameters)
Place
place() lets you position a widget either with absolute x,y coordinates, or relative to another widget.
Syntax : widget_name.place(parameters)
Grid
grid() positions widgets in a two dimensional grid of rows and columns similar to a dataframe.
Syntax : widget_name.grid(parameters)