HANDLING STRINGS
String is a collection of characters. Strings can be created by enclosing characters inside a single quote or double-quotes. Even triple quotes can be used in Python but generally used to represent multiline strings
Python does not have a character data type, a single character is simply a string with a length of 1.
Accessing Characters in a String
As we know, string is a collection of characters and individual character can be accessed by its position called index. Square brackets can be used to access elements of the string. The index of the first character (from left) in the string is 0 and the last character is n-1 where n is the length of the string.

Square brackets can be used to access elements of the string. If we give index value out of this range then we get an Index Error. The index must be an integer (positive, zero or negative).
Example:
>>>String1="PYTHONFORALL"
>>> String1[3] >>>String1[-3]
'H' #returns index 3 position 'A' #value returned using backward indexing
Concatenation
Concatenation means to join two values. In Python, + symbol is used to concatenate the strings.
Example:
>>>name="Rhea"
>>>msg="Hello "
>>>print(msg+name)
'Hello Rhea' #concatenated string
Repetition / Replication
Replication can be performed by using * operator between the string. It will repeat the string n times, where n is the integer provided.
Example:
>>>name="PythonForAll"
>>>print(name*4)
'PythonForAllPythonForAllPythonForAllPythonForAll' #Replication
Slicing
At times, we need to extract a subpart from the string and this process is called slicing. Slicing operation will return few characters extracted from the main string on the basis of provided index values.
Example:
>>>name="PythonForAll"
>>>print(name[0:6])
'Python' #Returns string from index position 0 to 5(6-1)
Note: Forward indexing and back indexing, both can be used
>>>name="PythonForAll"
>>>print(name[-6:-2])
'ForA' #Returns string from index position -6 to -3
Note: Step value can also be provided, if we can to skip some characters in between in uniform order.
>>>name="PythonForAll"
>>>print(name[1:8:2])
''yhno'' #Returns string from index position 1 to 7 by skipping to every 2nd position
>>>name="PythonForAll"
>>>print(name[::-1])
'llAroFnohtyP' #Returns string in reverse order
Hopefully, concept of slicing is clear. Practice with more variations in Do It Yourself section.
Membership
In Python, in and not in are two membership operators to find the appearance of a substring inside the string. If substring is found then, in operator returns True else it returns False. not in works exactly opposite of in operator.
Example:
>>>name="PythonForAll"
>>>on in name
True #returns True as 'on' is there in the string
>>>name="PythonForAll"
>>>All not in name
True #returns True as 'on' is there in the string
String Functions
Python is loaded with various functions which can be used to manipulate the string values. List of commonly used string functions are given below:
Let us understand the concept by studying few examples
Example 1: To create a menu based program with 3 options:
1. Input a string and obtain a new string by converting the inputted string into an uppercase.
2. Input a string and obtain a new string by converting the inputted string into an lowercase.
Code
Output
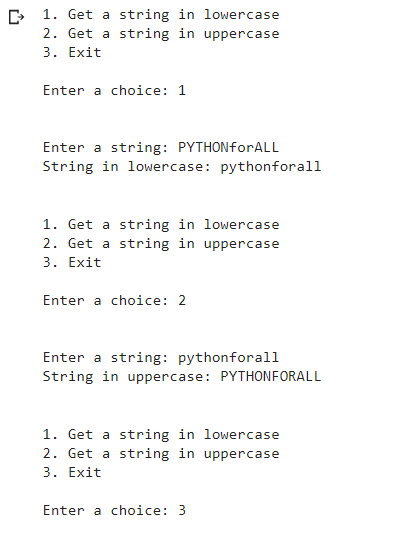
Example 2: Input string and obtain a new string by toggling the characters of the inputted string; display the new string.
Code
Output
Example 3: Input a string; check whether the inputted string is Palindrome or not (without using slice)
Code
Output

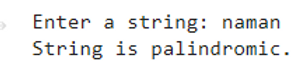