PYTHON COLLECTIONS
Python Collections
There are four collection data types in the Python programming language:
-
LIST is a collection which is ordered and changeable. Allows duplicate members.
-
TUPLE is a collection which is ordered and unchangeable. Allows duplicate members.
-
SET is a collection which is unordered, unchangeable, and unindexed. No duplicate members.
-
DICTIONARY is a collection which is ordered* and changeable. No duplicate members. Set items are unchangeable, but you can remove and/or add items whenever you like.
As of Python version 3.7, dictionaries are ordered. In Python 3.6 and earlier, dictionaries are unordered.
When choosing a collection type, it is useful to understand the properties of that type. Choosing the right type for a particular data set could mean retention of meaning, and, it could mean an increase in efficiency or security.
Code
Output

LIST
The data category list is an ordered sequence that contains one or more elements and is changeable. A list can contain more elements than a string, which can only contain letters. elements of several data kinds, including integer, float, and a list, text, or even a tuple. Using a list is a highly useful assemble pieces with varying data kinds. List components are separated by comma and contained in square brackets.
Example :-
#This List contains numbers (int)
>>> list1 = [1 , 2 , 3 , 4 , 5]
>>> print(list1)
output : [1 , 2 , 3 , 4 , 5]
#This List contains alphabets (string)
>>> list2 = ['a' , 'b' , 'c' , 'd' , 'e']
>>> print(list2)
output : ['a' , 'b' , 'c' , 'd' , 'e']
#This List contains mixed data types (string + int)
>>> list3 = ['a' , 1 , 'b' , 2 , 'c' , 3 ]
>>> print(list3)
output : ['a' , 1 , 'b' , 2 , 'c' , 3 ]
#This List is a Nested List which contains mixed data types (string + int)
>>> list4 = [ ['a' , 1] , ['b' , 2] , ['c' , 3] ]
>>> print(list4)
output : [ ['a' , 1] , ['b' , 2] , ['c' , 3] ]
Accessing Elements
A value called index is used to retrieve each element in a list.
The value of the first index is 0, the second index is 1, and so on. The list's elements have index values starting from 0 in increasing order.
Use square brackets with the element's index [] value to access it. Additionally, we can access elements from the end of the list by using negative indexing starting from -0.
Example :-
#Initialing a list named list1
>>> list1 = [1,3,5,7,9,11]
#Returns first element of list1
>>> list1[0]
output : 1
#Returns fourth element of list1
>>> list1[3]
output : 7
#Out of range index value for the list returns error
>>> list1[15]
output : IndexError: list index out of range
#An expression resulting in an integer index
>>> list1[1+4]
output : 11
#Return first element from right
>>> list1[-1]
output : 11
#Length of the list1 is assigned to n
>>> n = len(list1)
>>> print(n)
output : 6
#Get the last element of the list1
>>> list1[n-1]
output : 11
#Get the first element of list1
>>> list1[-n]
output : 1
Note :- Lists are mutable in Python
In Python, lists are mutable. Which means that the elements of the list can be changed after it has been created.
Example :-
#Create a List named list1
>>> list1 = ['Football ', 'Basketball' , 'Cricket' , 'Tennis']
#Change/override the second element of list1
>>> list1[1] = 'Handball'
#Print the modified list
>>> list1
output : ['Football ', 'Handball' , 'Cricket' , 'Tennis']
List Operations
1. Concatenation
Concatenation refers to joining or merging , In Python, we can concatenate 2 or more lists to create a single combined list.
Example :-
#Create a List named list1
>>> list1 = ['Football ', 'Basketball' , 'Cricket' , 'Tennis']
#Create another List named list2
>>> list2 = [1 , 2 , 3 , 4 ]
#Concatenate both the lists to form a new list
>>> list3 = list1 + list2
#Print the modified list
>>> list3
output : ['Football ', 'Handball' , 'Cricket' , 'Tennis' , 1 , 2 , 3 , 4]
Note :- Only a List can be concatenated with another List , a single element or variable cannot.
2. Repetition/ Replication
We can replicate a list 'n' number of times using repetition operator by using "*" .
Example :-
#Create a List named list1
>>> list1 = ['Hey ', 'Hi' ]
#Replicate the List
>>> list1 * 3
output : ['Hey ', 'Hi' , 'Hey ', 'Hi' , 'Hey ', 'Hi' ]
3. Membership
Membership operators 'in' or 'not in' look out for the presence of the given element inside the list.
Example :-
#Considering the given list:
>>> list1 = ['Football ', 'Basketball' , 'Cricket' , 'Tennis']
>>> 'Cricket' in list1
Output : True
>>> 'Squash' not in list1
Output : True
4. Slicing
Slicing means extracting a range of elements from the existing list. It is the most common and useful technique used by the programmers.
Example :-
#Considering the given list:
>>> list1 = ['Football ', 'Basketball' , 'Cricket' , 'Tennis', 'Kho Kho', 'Volleyball', 'Skating', 'Badminton']
# Displaying items from indexes 3 to 5
>>>list1[3 : 6]
Output : ['Tennis', 'Kho Kho', 'Volleyball']
#Note: It is always upper limit -1 i.e. 6-1=5 in the above example.
#Skiping the values in slicing. Here, 2 is used as skipping value.
>>>list1[1 : 6 : 2]
Output : [Basketball' , 'Tennis', 'Volleyball']
#Using backward indexing
>>>list1[-5 : -2]
Output : ['Tennis', 'Kho Kho', 'Volleyball']
#Using backward indexing and printing right to left
>>> list1[ -3 : -7 :-1]
Output : ['Volleyball', 'Kho Kho', 'Tennis', 'Cricket']
List Functions
Run the code yourself
DICTIONARY
Dictionary is another data type in python to hold sequence of values, but it's structure is different i.e. 'key : value' pairs. Dictionary can be created using the dict() or curly braces '{ }'.
Some characteristics of dictionary are:
-
A dictionary is an unordered set of 'key:value', means not a sequence
-
Values are indexed by keys, not numbers
-
Keys must be unique
-
Dictionaries are mutable i.e. their values can be changed in place
-
Internally they are stored as mapping
Example :-
#Creating a dictionary- here Names are keys and marks are values
>>> student={'Raghav': 89, 'Nitya': 91, 'Asif': 88}
>>> print(student)
output : {'Raghav': 89, 'Nitya': 91, 'Asif': 88}
# Creating an empty dictionary
>>> dict_marks={}
or
>>> dict_marks=dict()
#Creating nested dictionary
>>> student={'Raghav':{'Eng':78, 'Maths':87}, 'Nitya':{'Eng':78, 'Maths':87}}
>>>print(student)
Output : {'Raghav': {'Eng': 78, 'Maths': 87}, 'Nitya': {'Eng': 78, 'Maths': 87}}
Working with Dictionaries
1. Accessing a value from dictionary
Unlike list, dictionary values are fetched by keys here.
Example :-
#Initialing a dictionary named student
>>> student={'Raghav': 89, 'Nitya': 91, 'Asif': 88}
>>>print(student['Raghav'])
output : 89
2. Accessing keys
keys() function is used to fetch the keys from the dictionary.
Example :-
>>>student={'Raghav': 89, 'Nitya': 91, 'Asif': 88}
>>>student.keys()
output : dict_keys(['Raghav', 'Nitya', 'Asif'])
3. Accessing values
values() function is used to fetch the keys from the dictionary.
Example :-
>>>student={'Raghav': 89, 'Nitya': 91, 'Asif': 88}
>>>student.values()
output : dict_values([89, 91, 88])
4. Accessing items
items() function returns the list with all dictionary keys with values. The pairs from the dictionary are stored in the form of tuple.
Example :-
>>>student={'Raghav': 89, 'Nitya': 91, 'Asif': 88}
>>>student.items()
output : dict_items([('Raghav', 89), ('Nitya', 91), ('Asif', 88)])
5. Membership
Membership operator 'in' and 'not in' helps to know the existence of a particular key in te dictionary. Note, that membership operators fetch keys only.
Example :-
>>>student={'Raghav': 89, 'Nitya': 91, 'Asif': 88}
>>>'Nitya' in student
Output: True
6. Traversing a dictionary
Traversing means fetching the dictionary items one by one. To traverse, we need to iterate hence using loop.
Example :- Method 1
Example :- Method 2
7. Adding a new element to Dictionary
A new element can be added to the dictionary using assignment operator '='. But ensure that key should be unique otherwise existing key will get updated.
Example :-
>>>student={'Raghav': 89, 'Nitya': 91, 'Asif': 88}
>>>student['Pihu']=90
>>>print(student)
Output: {'Raghav': 89, 'Nitya': 91, 'Asif': 88, 'Pihu':90}
8. Updating existing element in a Dictionary
This is similar to what we did while adding a new element, if the key already exist it will be replaced by the new value.
Example :-
>>>student={'Raghav': 89, 'Nitya': 91, 'Asif': 88}
>>>student['Pihu']=94
>>>print(student)
Output: {'Raghav': 89, 'Nitya': 91, 'Asif': 88, 'Pihu':94}
9. Deleting element from a Dictionary
For removing key:value pair from the dictionary del statement is used.
Example :-
>>>student={'Raghav': 89, 'Nitya': 91, 'Asif': 88}
>>>del student['Asif']
>>>print(student)
Output: {'Raghav': 89, 'Nitya': 91}
#If a non existing key is passed then, python give KeyError
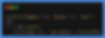.png)
Output:
Key= Raghav and Value= 89
Key= Nitya and Value= 91
Key= Asif and Value= 88

Output:
Key= Raghav and Value= 89
Key= Nitya and Value= 91
Key= Asif and Value= 88
Dictionary Functions
Run the code yourself
Tuple
Tuple is an ordered collection of elements from various data types, such as integer, float, string, list, or even another tuple. It can be created using the tuple() or round brackets ( ).
Example :-
#Creating a tuple with integers
>>> tuple1 = (89, 91, 88)
>>> print(tuple1)
output : ( 89, 91, 88)
# Creating an empty tuple
>>> tuple_marks=()
or
>>> tuple_marks=tuple()
#Creating tuple with mixed values
>>> tuple2=(23,45,'Delhi')
>>>print(tuple2)
Output : (23, 45, 'Delhi')
#Creating nested tuple
>>> tuple3=(23,45,(4,6),[2,-30])
>>>print(tuple3)
Output : (23, 45, (4, 6), [2, -30])
Tuple is Immutable
It means that the elements of a tuple cannot be changed after it has been created.
An attempt to do this would lead to an error.
Example :-
>>>tuple1=(2,4,6)
>>>tuple1[1]=40
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
tuple1[1]=40
TypeError: 'tuple' object does not support item assignment
Creating tuple with single element
If there is only a single element in a tuple then the element should be followed by a comma. If we assign the value without comma it is treated as integer.
Example:-
>>>single_tuple=(23)
>>>type(single_tuple)
Output : <class 'int'>
Correct way of doing it :
>>>single_tuple=(23,)
>>>type(single_tuple)
Output : <class 'tuple'>